This post is for myself to remember how to build Django project.
I did follow the tutorial from the JustDjango Learn website free tutorial.
And this is the fourth tutorial named Getting Started With Django.
I am not going over all the details and descriptions for each part. There are good explanations on video of the JustDjango Learn. So, visit their site and try their tutorials if you need more details. Also, the orders of this post and their video might be different because I put things first what I think should come first.
I am doing this on Windows 10 with just Windows PowerShell. Not using virtual machines at all.
1. Create super user
- in case, you are out of virtual environment, activate it.
- on terminal, type below command to create super user
python manage.py createsuperuser
2. Add models to admin page
- first run server
- open up the browser and go to localhost:8000/admin
- login with your super user
- you will see just “Groups” there.
- go back to VSCode
- open admin.py under leads folder
- add below code and save the file
from .models import Agent, Lead, User
admin.site.register(Agent)
admin.site.register(Lead)
admin.site.register(User)
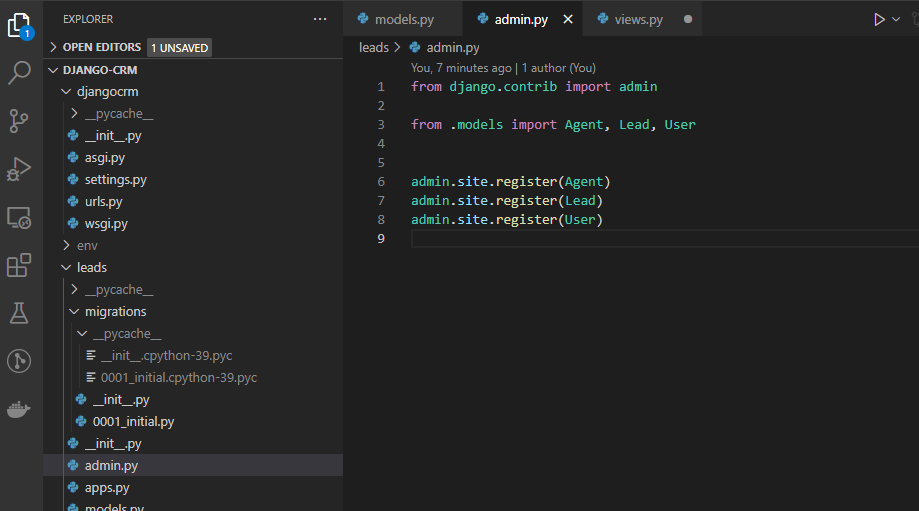
- refresh browser
- now you will see more on your admin page
- you can play around to add/delete/update
3. Views and Urls(“Hello World”)
- go to views.py under leads folder
- add below code and save the file
from django.http import HttpResponse
def home_page(request):
return HttpResponse("Hello world")
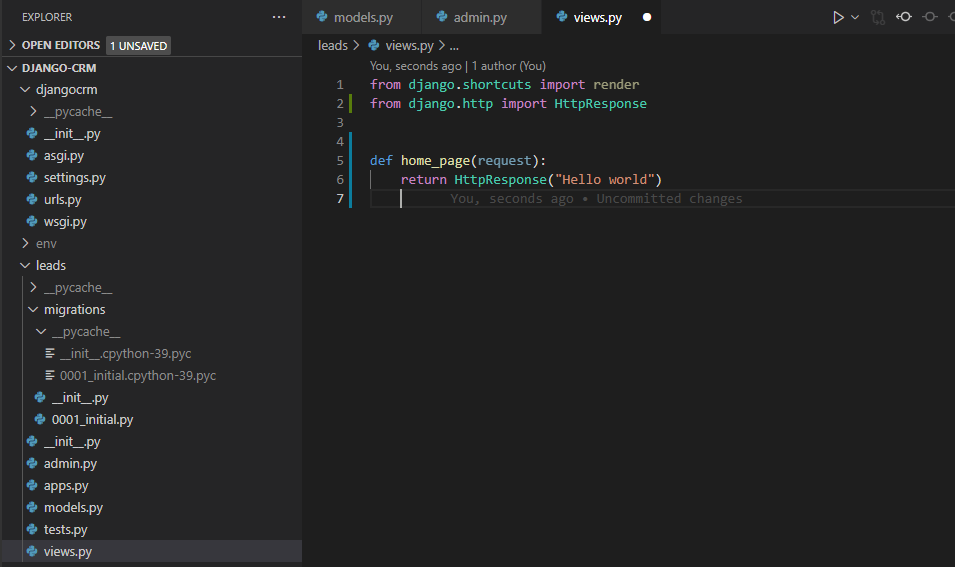
- go to urls.py under the djangocrm folder
- import home_page from leads app and add path like code below
from leads.views import home_page
urlpatterns = [
path('admin/', admin.site.urls),
path('', home_page)
]
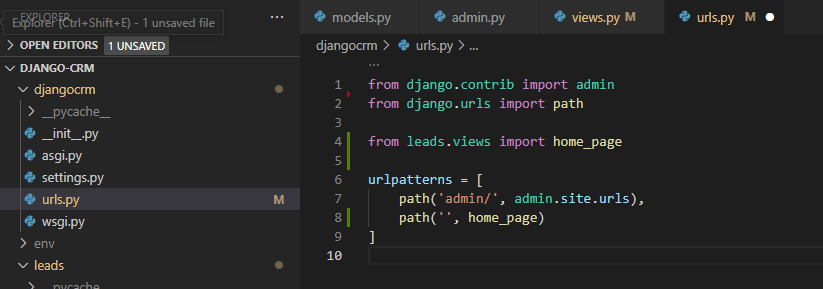
- go to your browser, go to localhost:8000
- You will see “Hello World”
4. Templates
- There are two ways of using templates, global templates folder and templates folder inside app.
- I will use the first one in this post, and if you want to compare them, please visit JustDjango Learn.
- Create the folder name “templates” under root folder.
- Create the file name “home_page.html”
- Write or copy the code below in home_page.html file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>test</title>
</head>
<body>
<h1>Hello World</h1>
</body>
</html>
- Save the file
- Go to settings.py under the djangocrm folder.
- Find the “TEMPLATES”
- under “‘DIRS’” write or copy codes below.
BASE_DIR / 'templates'
- Go to views.py under leads folder.
- Edit after the return as below code.
render(request, "home_page.html")
- Save the file
- refresh browser
- you will see the change of hello world bigger
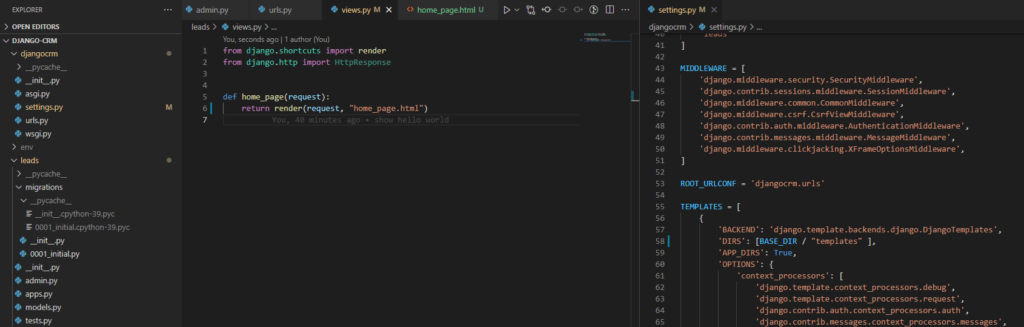
5. Context
- it is the concept that parse information into the templates
- go to views.py
- import Lead and add some codes like below
from .models import Lead
def home_page(request):
leads = Lead.objects.all()
context = {
"leads": leads
}
return render(request, "home_page.html", context)
- go to home_page.html
- add some code below hello world
<ul>
{% for lead in leads %}
<li>{{ lead }}</li>
{% endfor %}
</ul>
- save both views.py and home_page.html
- go to browser and refresh
- you will see the lists of leads if you have added some
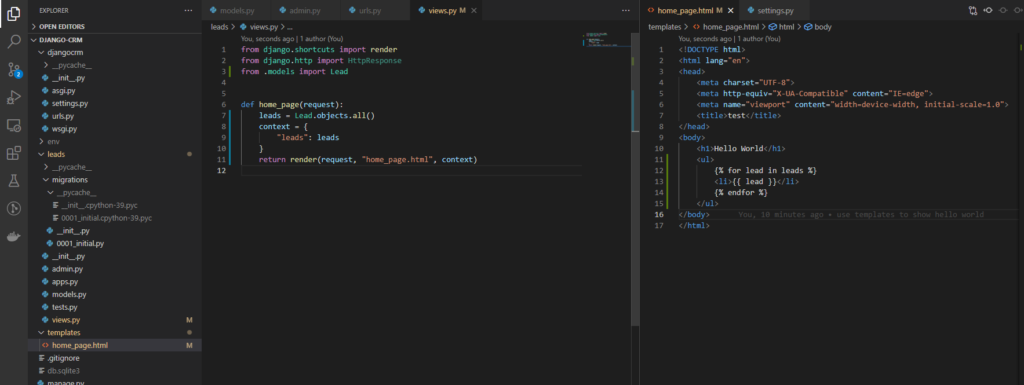
6. Restructure Urls
- create new file name urls.py under leads folder
- add the code below
from django.urls import path
from .views import home_page
app_name = "leads"
urlpatterns = [
path('all/', home_page)
]
- go to urls.py under djangocrom folder
- delete home_page import
- import include along with path
- add path for leads
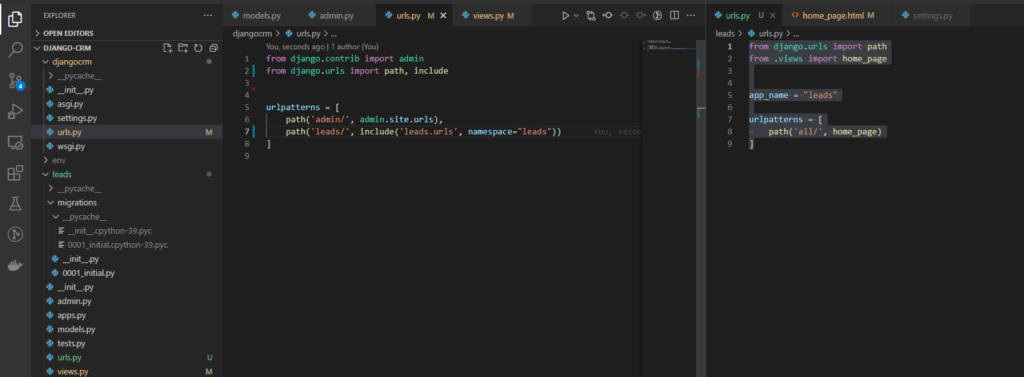
- save both urls.py files
- go to browser and try refresh, then you will get error.
- go to “localhost:8000/leads/all/”
- now you will see the hello world page.
Done for this post.