This post is for myself to remember how to build Django project.
I did follow the tutorial from the JustDjango Learn website free tutorial.
And this is the fourth tutorial named Getting Started With Django.
I am not going over all the details and descriptions for each part. There are good explanations on video of the JustDjango Learn. So, visit their site and try their tutorials if you need more details. Also, the orders of this post and their video might be different because I put things first what I think should come first.
I am doing this on Windows 10 with just Windows PowerShell. Not using virtual machines at all.
1. Rename/Restructure Urls
- rename home_page.html to lead_list.html
- go to views.py and rename the function and render page as lead_list
- go to urls.py and delete all/ at path.

2. add some style and edit the body on html
- add style code below in <head> below <title> on lead_list.html file
<style>
.lead {
padding-top: 10px;
padding-bottom: 10px;
padding-left: 6px;
padding-right: 6px;
margin-top: 10px;
background-color: #f6f6f6;
width: 100%;
}
</style>
- edit the body as code below
<h1>This is all of our leads</h1>
{% for lead in leads %}
<div class="lead">
{{ lead.first_name }} {{ lead.last_name }} Age: {{ lead.age }}
</div>
{% endfor %}
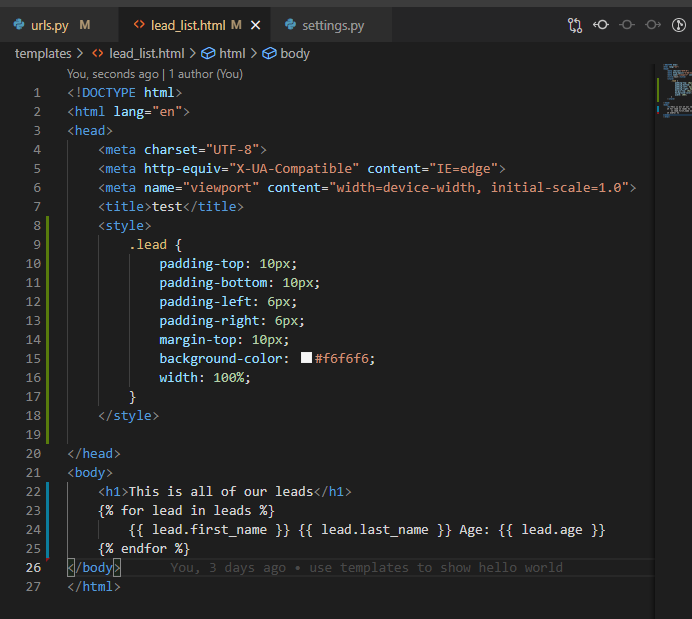
- save the files
- go to browser, and go to “localhost:8000/leads”
- you will see some changes.
3. Add lead detail view
- go to views.py
- add detail function code below.
def lead_detail(request, pk):
lead = Lead.objects.get(id=pk)
context = {
"lead": lead
}
return render(request, "lead_detail.html", context)
- go to urls.py
- add import and path of lead_detail
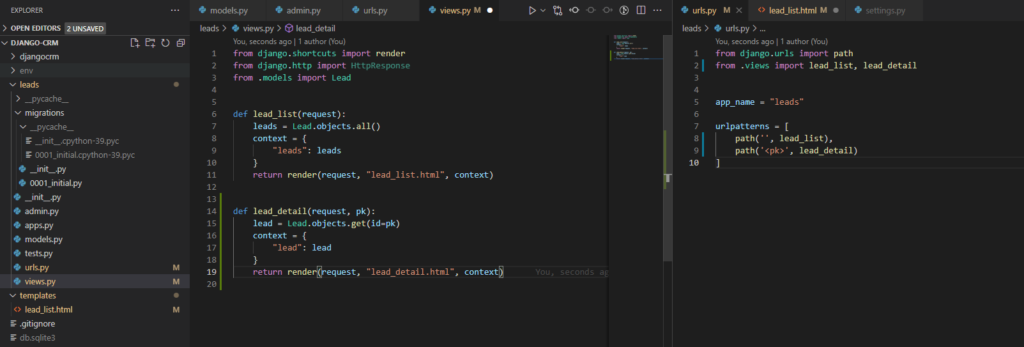
- create lead_detail.html file under template folder
- copy and paste the code below
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>test</title>
</head>
<body>
<h1>This is the details of {{ lead.first_name }}</h1>
<p>This person's age: {{ lead.age }}</p>
</body>
</html>
- save files
- go to browser and go to “localhost:8000/leads/1”
- you will see the new page with “here is the detail view”.
4. add button to go to detail page and go back button
- go to lead_list.html file
- add anchor tag for first and last name as code below.
<a href="/leads/{{ lead.pk }}/">{{ lead.first_name }} {{ lead.last_name }}</a>. Age: {{ lead.age }}
- save and go to “localhost:8000/leads” on your browser
- click the button.
- you might see the error page.
- that’s because of the / at the end (I guess)
- to fix this go to urls.py under leads folder.
- add / after <pk>
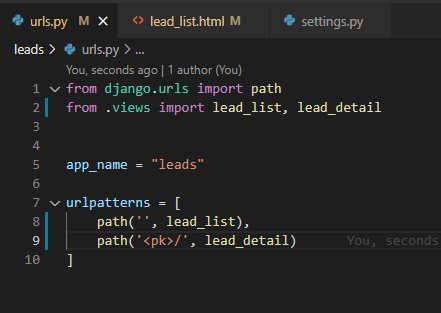
- save and go to your browser to check.
- you will see the detail page now.
- go to lead_detail.html file.
- add code below above the <h1>
<a href="/leads">Go back to leads</a>
<hr />
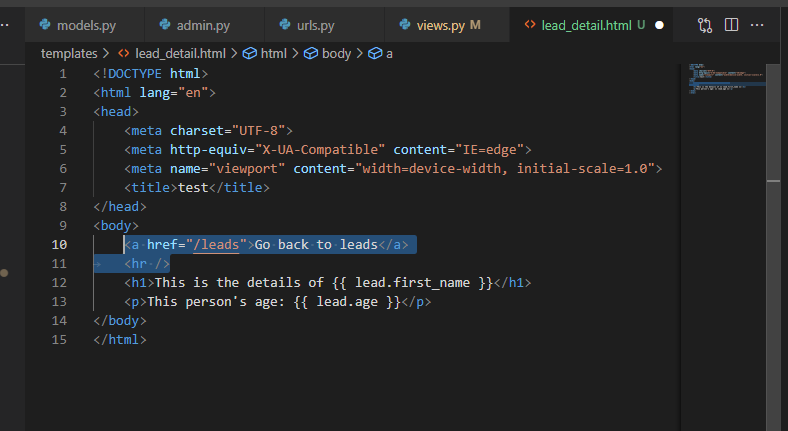
5. Create views and forms
- create function in views.py
def lead_create(request):
return render(request, "lead_create.html")
- create new html file lead_create.html and copy below code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>test</title>
</head>
<body>
<a href="/leads">Go back to leads</a>
<hr />
<h1>Create a new lead</h1>
</body>
</html>
- add create view on urls
- there are two ways.
urlpatterns = [
path('', lead_list),
path('<int:pk>/', lead_detail),
path('create/', lead_create)
]
urlpatterns = [
path('', lead_list),
path('create/', lead_create),
path('<pk>/', lead_detail)
]
- the reason why create needs to come first is the way python(maybe all other languages too) work is reading the code from the above. and <pk> is expecting the integer, but create is the string. so, force to set the pk as int, or let pk comes the last.
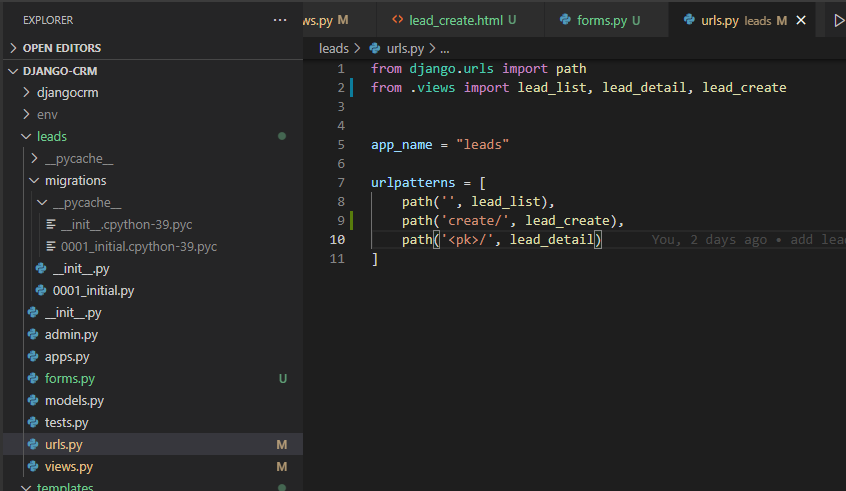
- create new file forms.py and copy below code
from django import forms
class LeadForm(forms.Form):
first_name = forms.CharField()
last_name = forms.CharField()
age = forms. IntegerField(min_value=0)
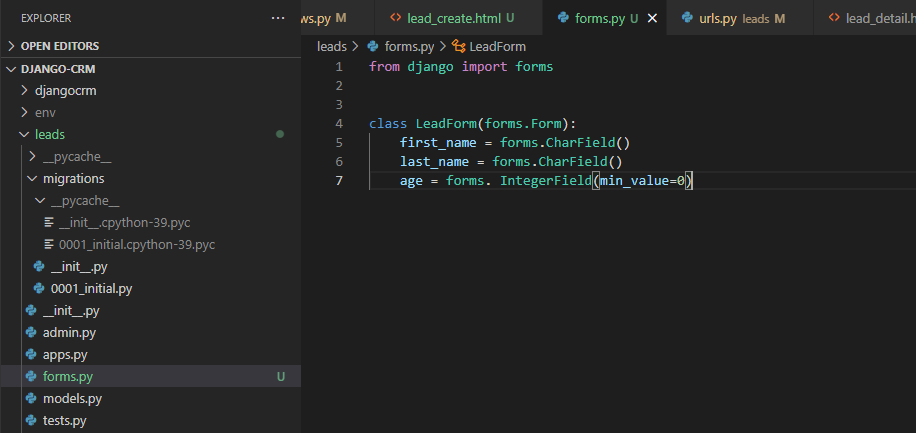
- import forms, agent, and redirect in views.py
- add forms under lead_create like below code. for the detail explanation, please visit their site.
form = LeadForm()
if request.method == "POST":
form = LeadForm(request.POST)
if form.is_valid():
first_name = form.cleaned_data['first_name']
last_name = form.cleaned_data['last_name']
age = form.cleaned_data['age']
agent = Agent.objects.first()
Lead.objects.create(
first_name=first_name,
last_name=last_name,
age=age,
agent=agent
)
return redirect("/leads")
context = {
"form": form
}
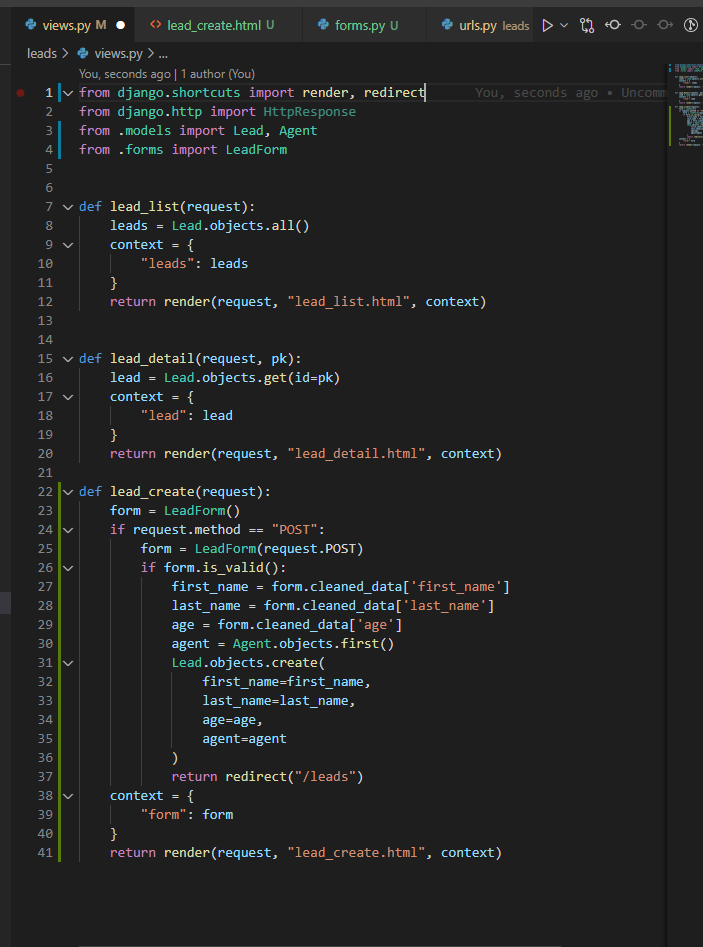
- add forms on html file
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Submit</button>
</form>
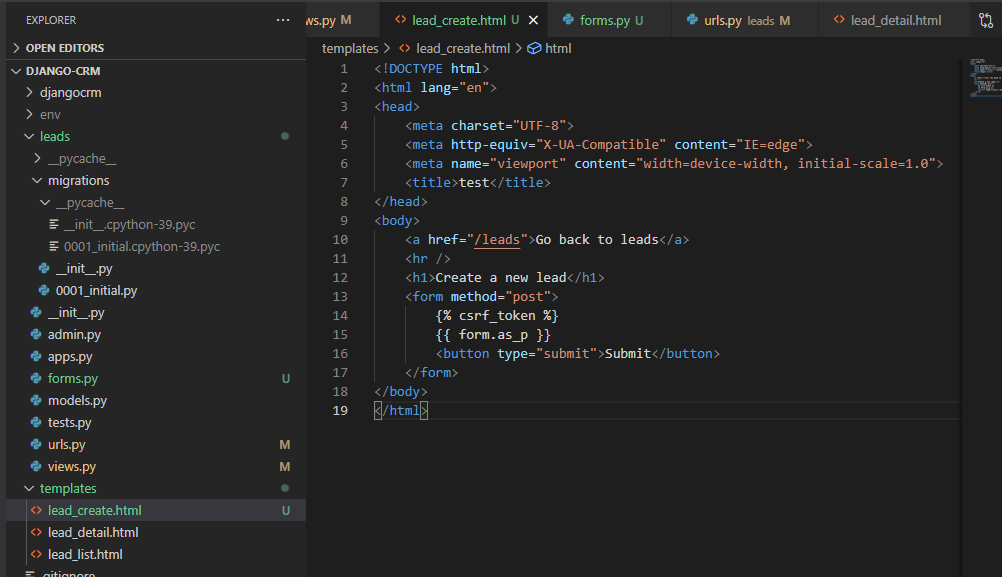
Done for this post.