This post is for myself to remember how to build Django project.
I did follow the tutorial from the JustDjango Learn website free tutorial.
And this is the fourth tutorial named Getting Started With Django.
I am not going over all the details and descriptions for each part. There are good explanations on video of the JustDjango Learn. So, visit their site and try their tutorials if you need more details. Also, the orders of this post and their video might be different because I put things first what I think should come first.
I am doing this on Windows 10 with just Windows PowerShell. Not using virtual machines at all.
1. configuration for deployment
- https://github.com/joke2k/django-environ
- install the above package
pip install django-environ
- add it to the requirements.txt
pip freeze > requirements.txt
- create .env file in djangocrm folder
- add debug as true and secret key from the settings.py
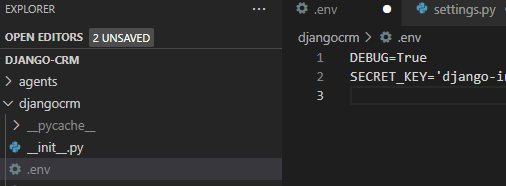
- go to settings.py
- you can copy the below code
- or add like below screenshot. you can copy them from the above github link
- the link above import and use os, but I and the video tutorial does not.
import environ
env = environ.Env(
# set casting, default value
DEBUG=(bool, False)
)
READ_DOT_ENV_FILE = env.bool('READ_DOT_ENV_FILE', default=False)
if READ_DOT_ENV_FILE:
environ.Env.read_env()
# False if not in os.environ because of casting above
DEBUG = env('DEBUG')
# Raises Django's ImproperlyConfigured
# exception if SECRET_KEY not in os.environ
SECRET_KEY = env('SECRET_KEY')
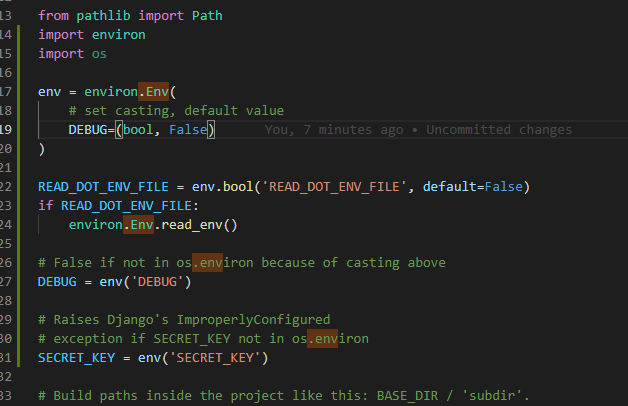
- and delete the original debug and secret_key from the settings.py
- create new file name .template.env
- and add debug and secret_key without values or dummies
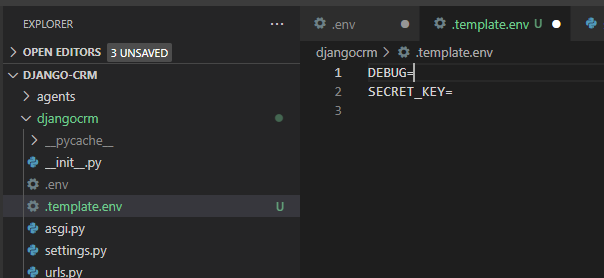
- this will be on the github instead .env file.
- save and try to run server(if you successfully run server, probably delete the terminal and reopen it to test)
- you will get the error like the below screenshot
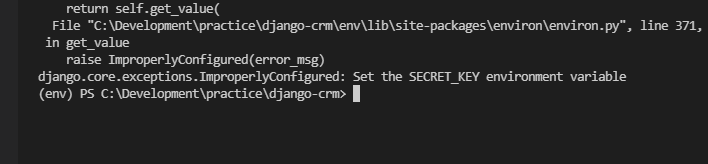
- To be able to run the server, type the below command to the console
export READ_DOT_ENV_FILE=True
- in powershell, it gives error about “export” term.
- so, the “export” command is for linux and mac
set READ_DOT_ENV_FILE=True
- “set” or “setx” commands are for cmd on windows
$env:READ_DOT_ENV_FILE="True"
- “$env:” command is for powershell
- now try to run server. it will work.
- How to set environment variables
2. set up postgresql
- first, install the postgresql
- after installed, or if you already have postgresql, check whether postgresql is running or not.
- to check, hit ctrl + r, and type servic.msc
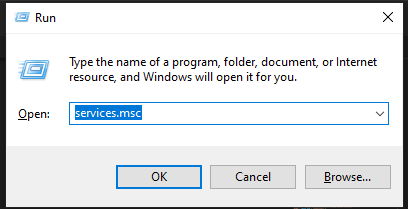
- find postgresql and check the status
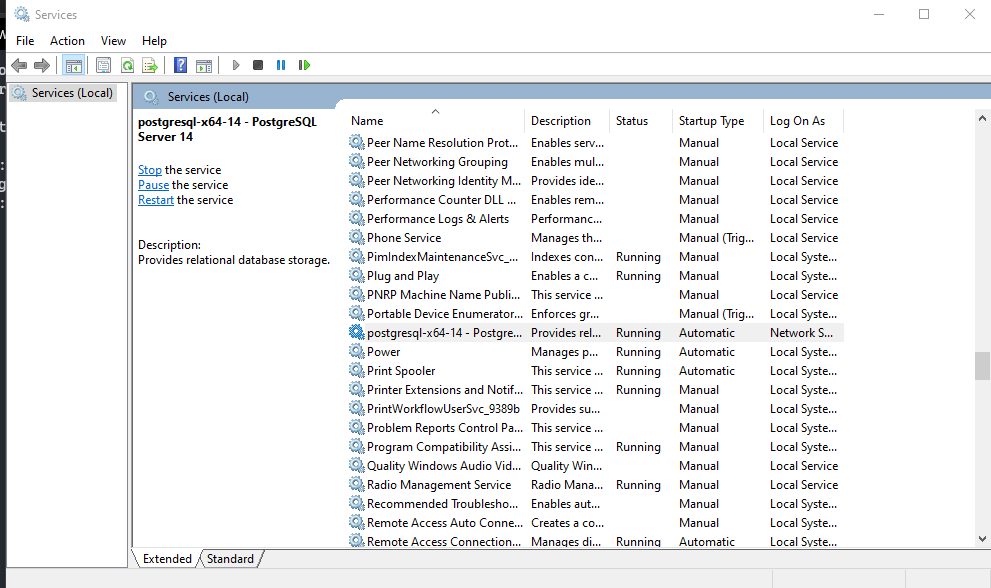
- optionally, to use postgresql graphically on windows, you can download pgadmin.
- you might already installed while installing postgresql
- in my case, I had issues/errors running postgresql
- basically, I didn’t add the data folder to the environment variables and didn’t create PGDATA.
- also, just in case, I initialized and restart the server.
- https://aeadedoyin.medium.com/getting-started-with-postgresql-on-windows-201906131300-ee75f066df78
- the above link is for my reference.
- install psycopg2
pip install psycopg2-binary
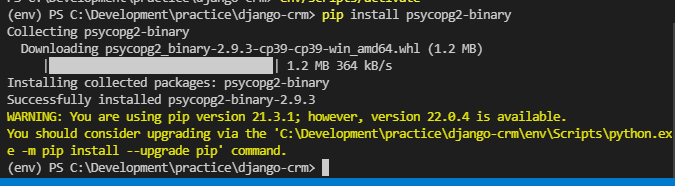
- add on requirements.txt
pip freeze > requirements.txt
- check the requirements.txt file
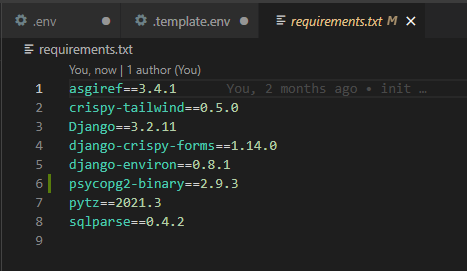
- create a new database on your console.
createdb djangocrm
- instead “djangocrm” type whatever you want to name the db.
- to create user on postgresql, the tutorial was typing below.
psql
- but in my case, to get into the postgresql shell on powershell, I need to type below.
psql postgres
- when you get to postgres sql, enter the commands below to create user.
CREATE USER admin WITH PASSWORD 'admin';
GRANT ALL PRIVILEGES ON DATABASE djangocrm TO admin;
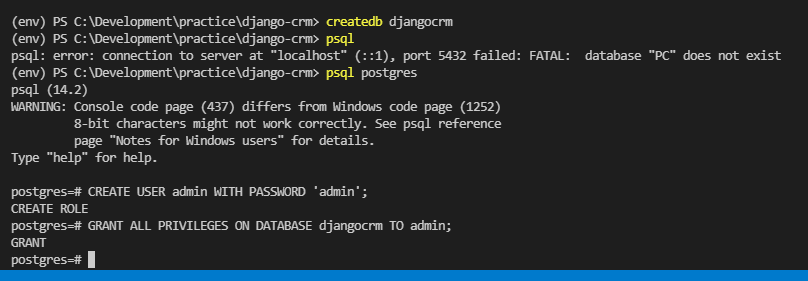
- type \q to exit from the postgresql console.
- go to settings.py
- edit the DATABASES like below
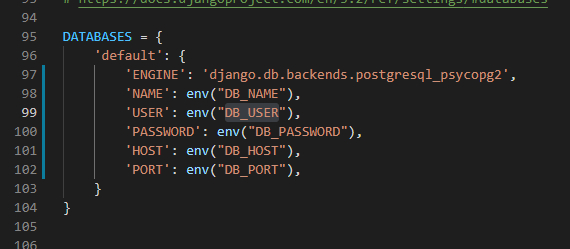
- go to .env file
- add about dbs
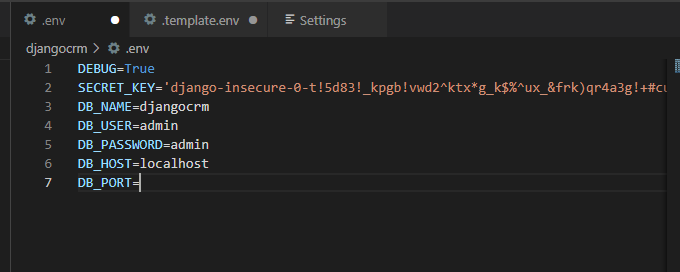
- and also on .template.env file without infos
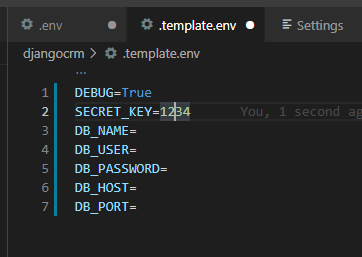
- save all the files
- try to run server
- you will get red messages because you just created new db. (in case you closed the vscode after the #1 tutorial, you need to set the env again.)
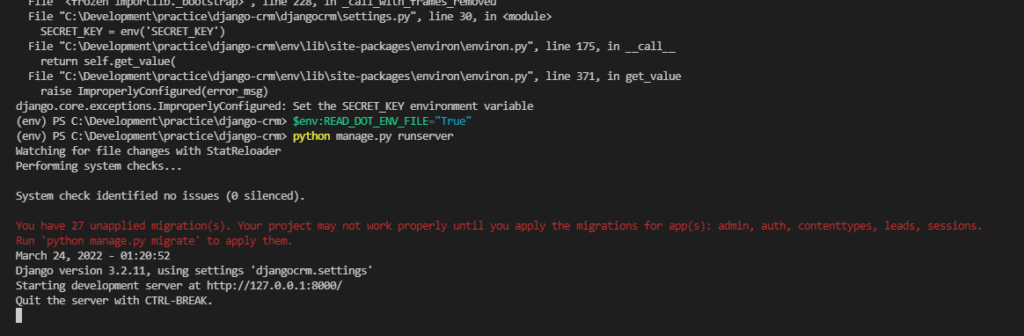
- stop the server, migrate and run server again to test
- run server, now you will have no issue to run server.
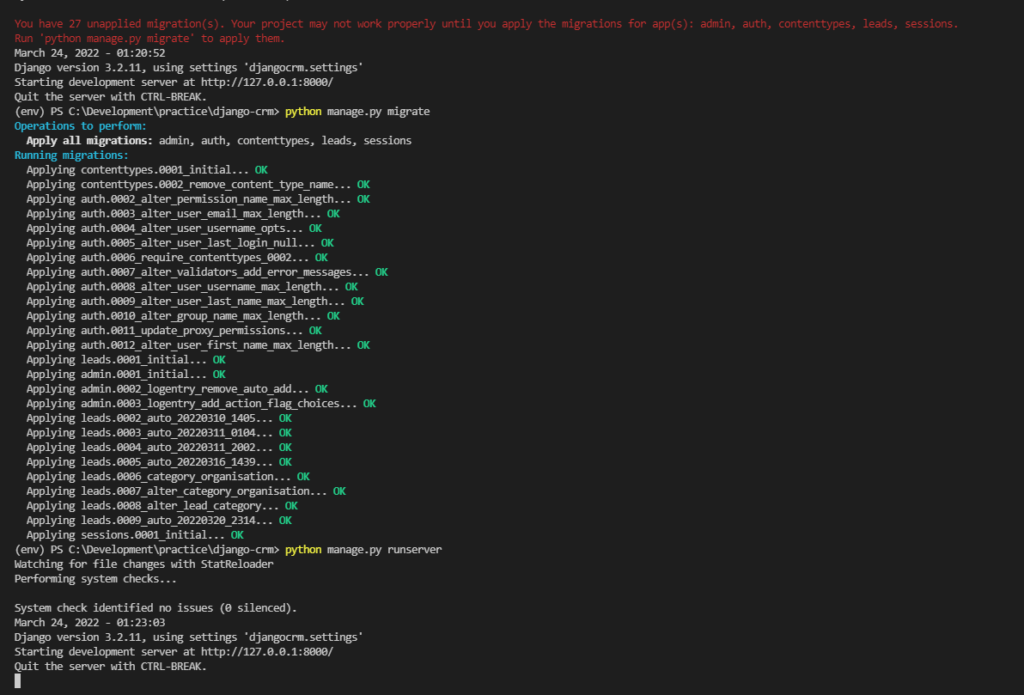
- now, we are no longer using sqlite db.
- since we created new db, we need to recreate the superuser
3. Whitenoise
- there are two options to store the static files.
- one is using the service like digital ocean or AWS s3
- another is using python application package name whitenoise.
- in this tutorial we will use whitenoise.
- go to WhiteNoise documentation to see how to start
- install whitenoise and add to requirements.txt
pip install whitenoise
pip freeze > requirements.txt
- add whitenoise on MIDDLEWARE after the SecurityMiddleware
- in the video, he added on top, but the document says after the SecurityMiddleware.
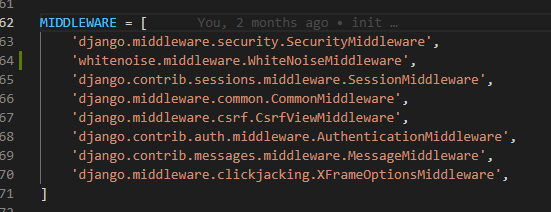
- add STATICFILES_STORAGE at the bottom
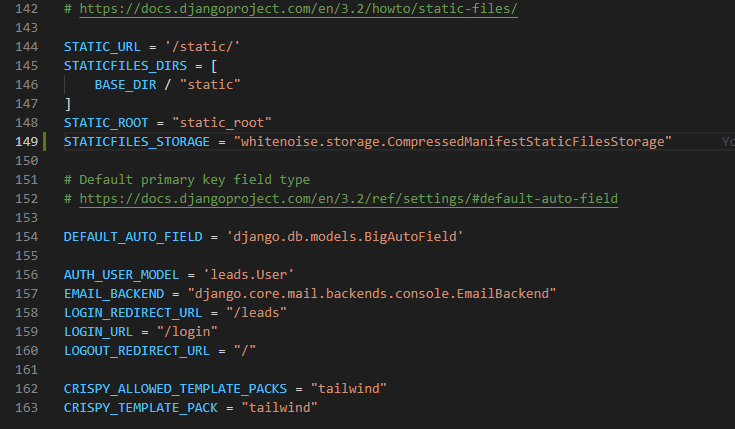
- type the command below to collect all the static files into one place.
python manage.py collectstatic
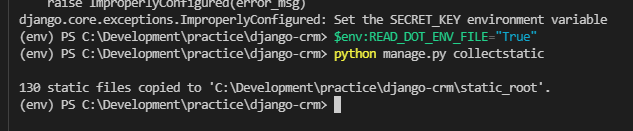
- add whitenoise on top of INSTALLED_APPS
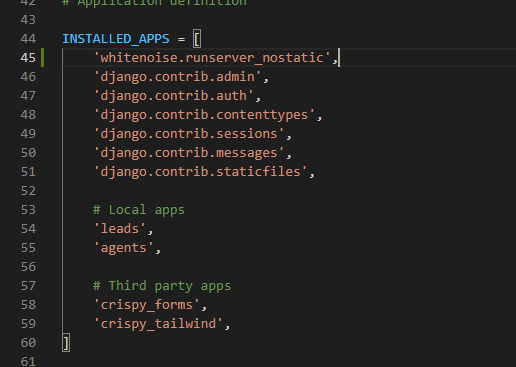
done for this post