This post is for myself to remember how to build Django project.
I did follow the tutorial from the JustDjango Learn website free tutorial.
And this is the fourth tutorial named Getting Started With Django.
I am not going over all the details and descriptions for each part. There are good explanations on video of the JustDjango Learn. So, visit their site and try their tutorials if you need more details. Also, the orders of this post and their video might be different because I put things first what I think should come first.
I am doing this on Windows 10 with just Windows PowerShell. Not using virtual machines at all.
1. built-in authentication source code provided by django
- to see the source code go to
- env -> bin -> lib/python -> site-packages -> django -> contrib -> auth -> views.py
- the above path is from the video, but my local has little different.
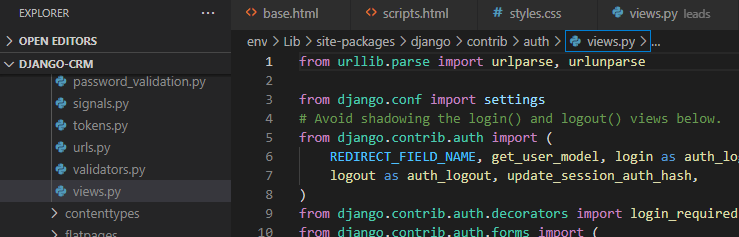
- for the description, please see their video about authentication
2. create login
- create registration folder under template
- create login.html file under registration folder
- write/copy code below
{% extends 'base.html' %}
{% block content %}
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Login</button>
</form>
{% endblock content %}
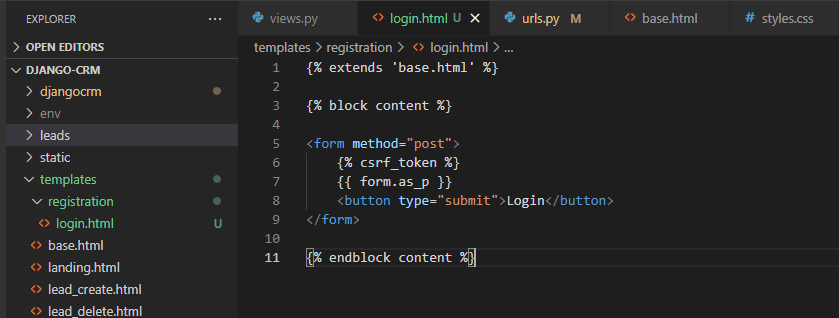
- go to urls.py under root project folder, djangocrm in my case
- import Loginview and add path for login like screenshot below
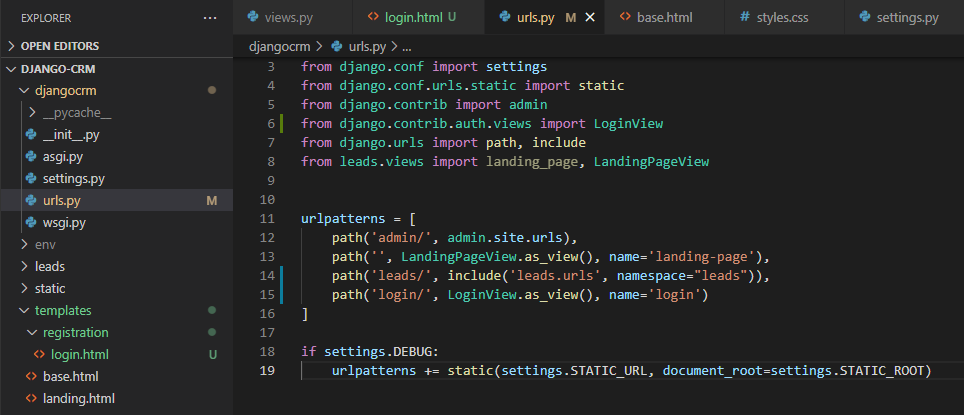
- save files and go to “http://localhost:8000/login/” to check if the login page shows
- since we have superuser already, you should be able to login with superuser
- if you try to login, you will see error like below screen
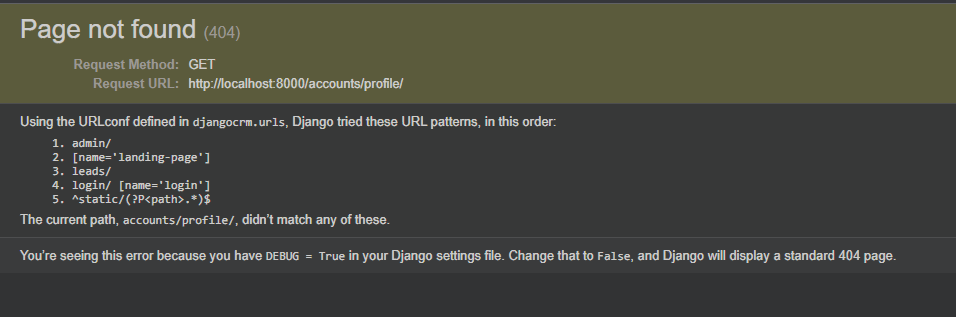
- this means you are logged in, but the url “http://localhost:8000/accounts/profile/” is defined as default by django after login
- to change the redirect page after login, go to settings.py
- add code below at the bottom
LOGIN_REDIRECT_URL = "/leads"
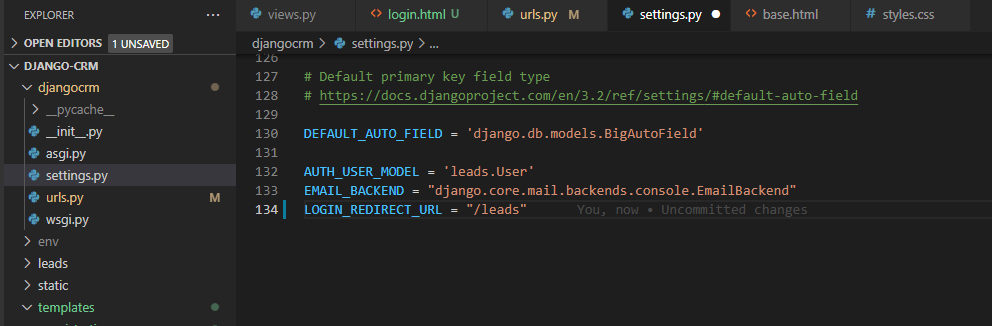
- after save the file, test to login again
- after you logged in, you don’t know you are logged in now.
- to show you are whether logged in or not, go to navbar.html
- add code like below screenshot
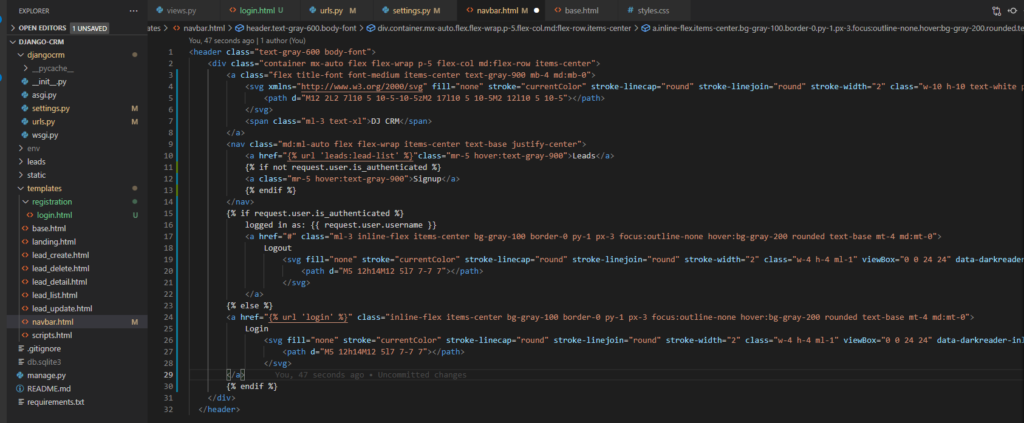
3. create logout
- go to urls.py under root project folder
- import LogoutView
- add path
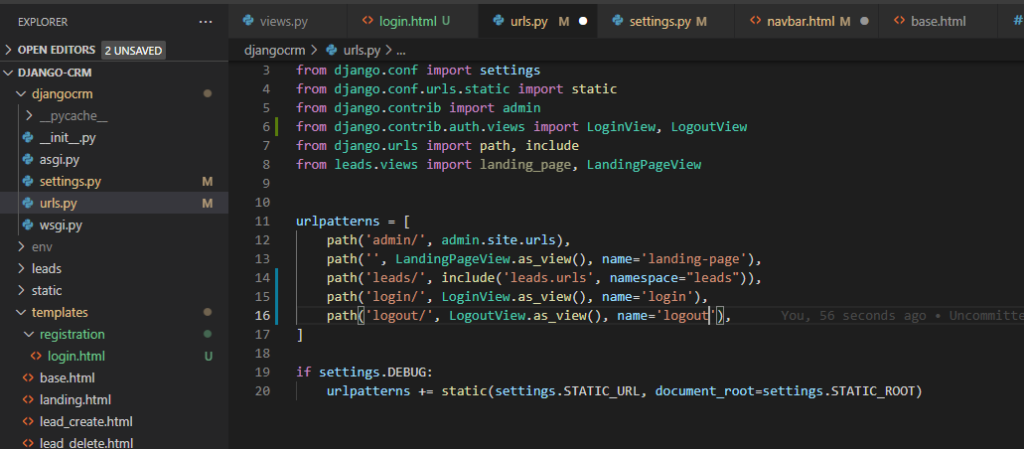
- go to navbar.html
- add href link for logout
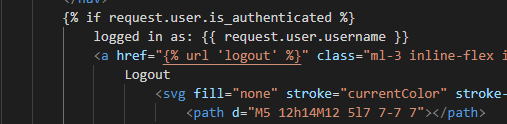
4. Create Signup
- there is no signup view in source code, so need to create our own
- create signup.html under registration folder
- copy content from login.html and just change “Login” to “Signup”
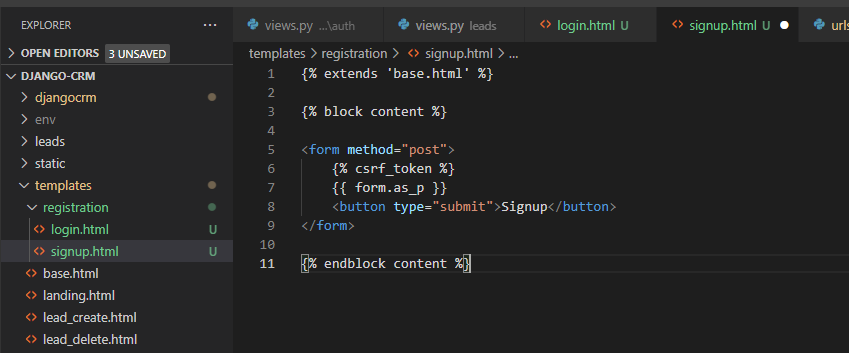
- go to views.py under leads folder
- import UserCreationForm
- add SignupView class
class SignupView(generic.CreateView):
template_name = "registration/signup.html"
form_class = UserCreationForm
def get_success_url(self):
return reverse("login")
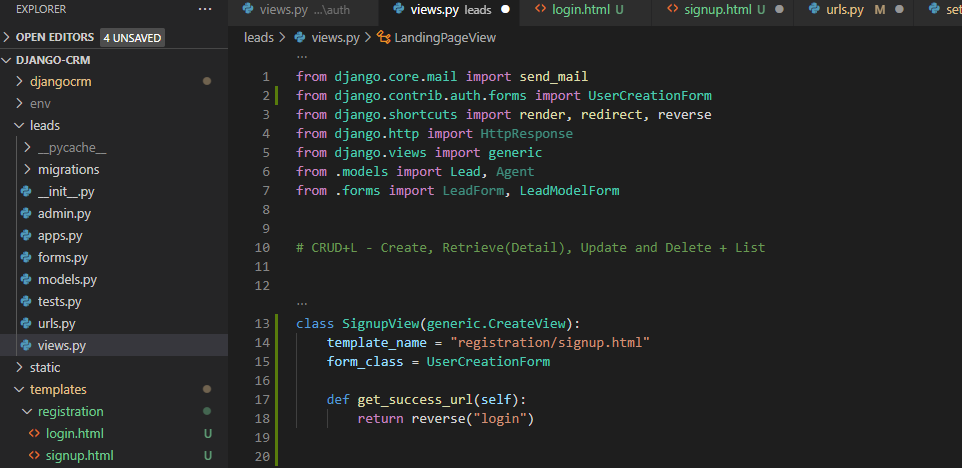
- go to urls.py under djangocrm folder
- import SignupView and add path
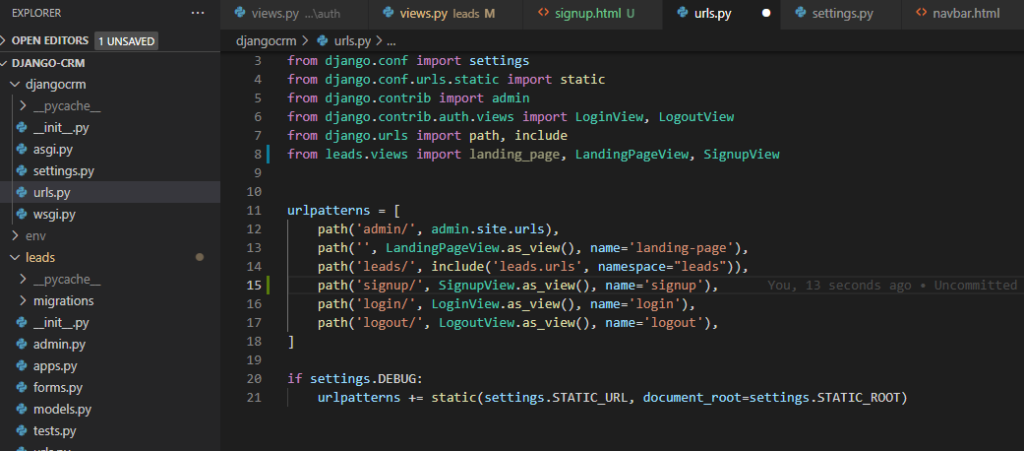
- go to navbar.html
- add href link for signup
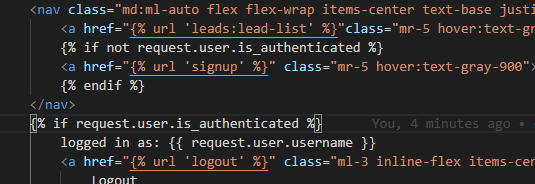
- save files, go to browser, if you are logged in, log out
- go to home or lead list page
- click signup button
- signup for test user
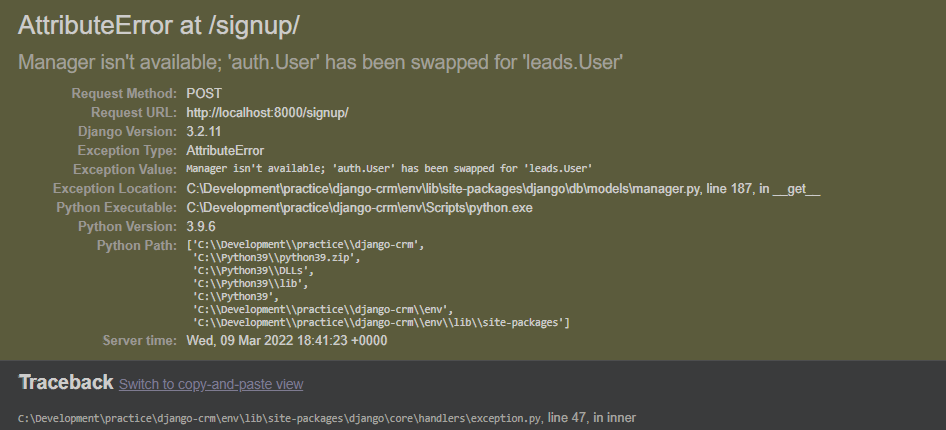
- you will see this kind of error
- this is because user is defined as built in django model user
- to make it custom model, go to forms.py under leads folder
- import some and add class there like below screenshot
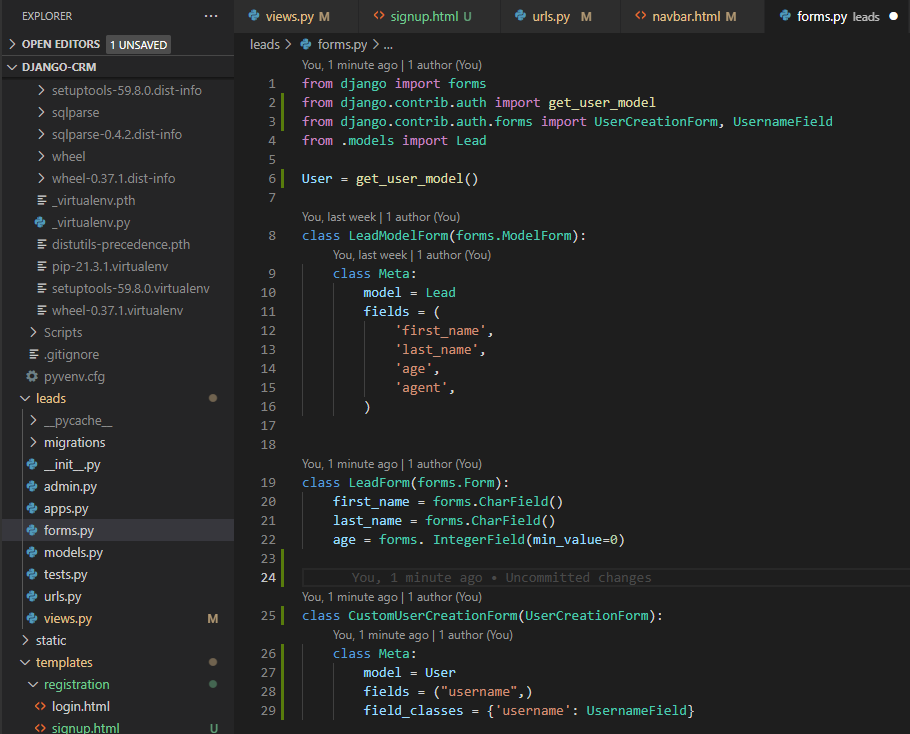
- go to views.py
- import CustomUserCreationForm from .forms
- and get rid of UserCreationForm
- replace UserCreationForm to CustomUserCreationForm on class
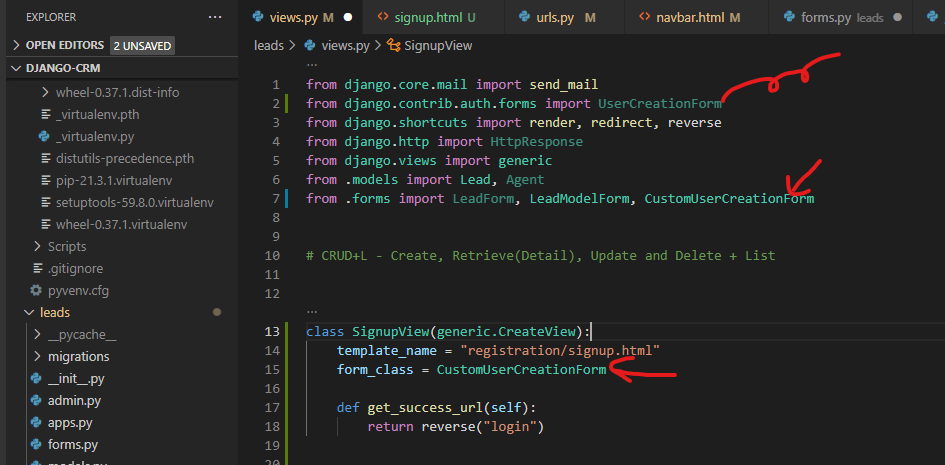
- save files and try to signup again and try login
- to see users logout from the test user, login again with superuser
- go to http://localhost:8000/admin/ and go to Users tab.
5. tests
- again, I am not going over all the details, for the description of each of them, please go to their site and watch the video.
- below steps are for when the projects getting bigger. and it is explained almost at the end of the video.
- create folder name tests
- create init file
- move tests.py into tests folder and rename it, and create another file
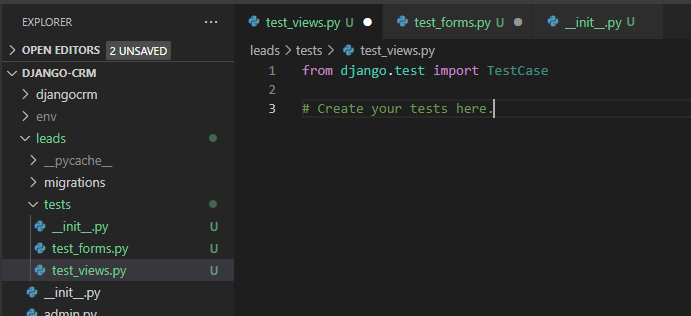
- write/copy code below on test_views.py
from django.test import TestCase
from django.shortcuts import reverse
# Create your tests here.
class LandingPageTest(TestCase):
def test_get(self):
response = self.client.get(reverse("landing-page"))
self.assertEqual(response.status_code, 200)
self.assertTemplateUsed(response, "landing.html")
- stop the server on console
- type “python manage.py test”
- see the result
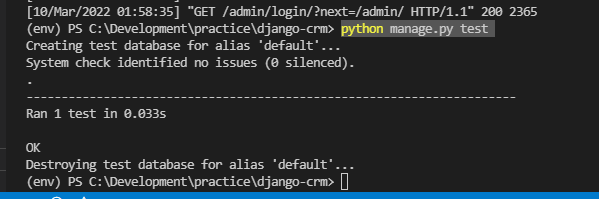
done for this post