This post is for myself to remember how to build Django project.
I did follow the tutorial from the JustDjango Learn website free tutorial.
And this is the fourth tutorial named Getting Started With Django.
I am not going over all the details and descriptions for each part. There are good explanations on video of the JustDjango Learn. So, visit their site and try their tutorials if you need more details. Also, the orders of this post and their video might be different because I put things first what I think should come first.
I am doing this on Windows 10 with just Windows PowerShell. Not using virtual machines at all.
This post
1. Styling references
- there are a lot of sites for styling. bootstrap, tailwind, tailblocks etc.
- we will use tailwind and tailblocks here.
- go to tailwind site, documents -> installation -> play CDN
- it is little different with the video.
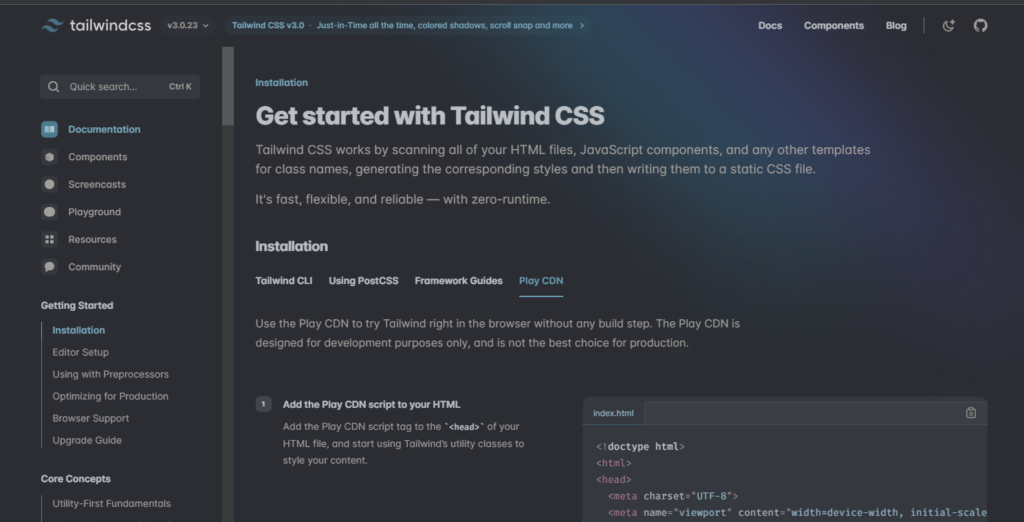
- copy the CDN script into bottom of head on base.html file.
- try some styling on body to check things are working property like below.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>test</title>
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body>
<div class="max-w-4xl mx-auto bg-gray-100">
{% block content %}
{% endblock content %}
</div>
{% include "scripts.html" %}
</body>
</html>
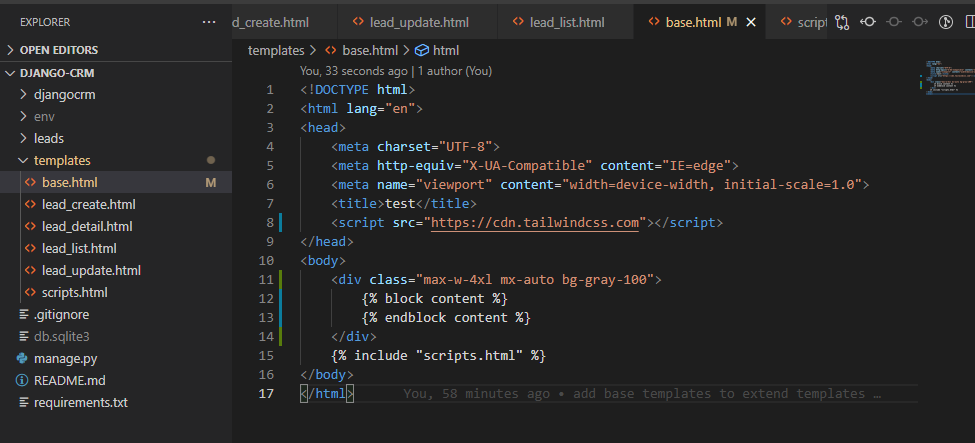
- if you check on your browser, you will see like the below screenshot.
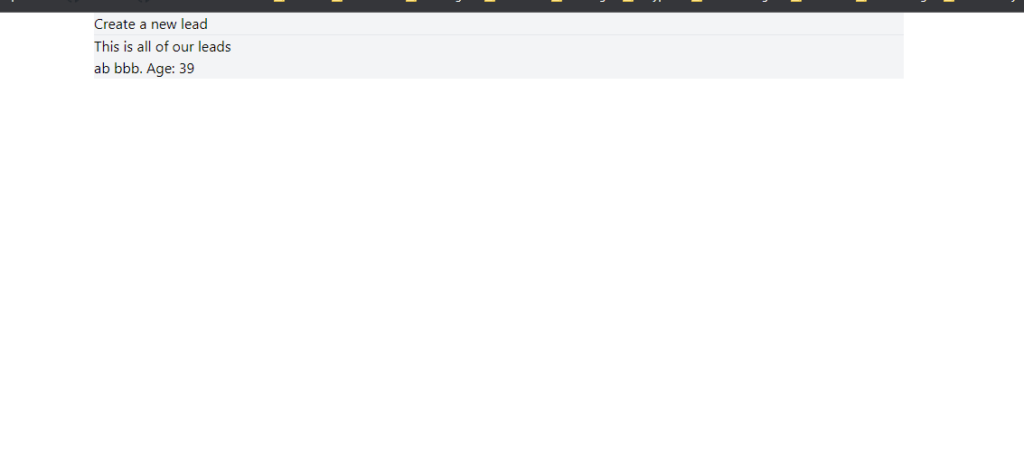
2. Using tailblocks to style navbar
- go to tailblocks site find header on the left sidebar
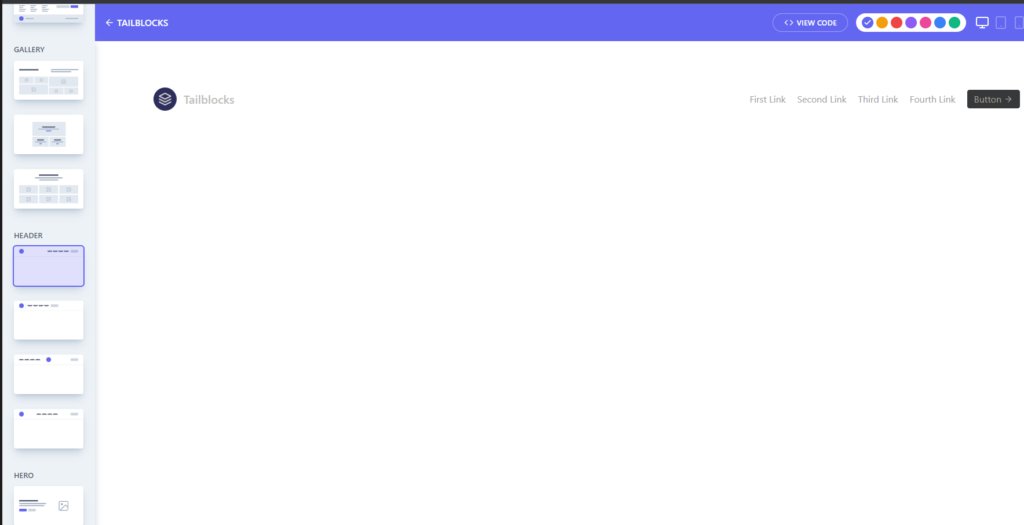
- choose one you like, and click view code and copy the entire code.
- create navbar.html file and paste the code
- add {% include “navbar.html” %} to body on base.html and edit some styles.
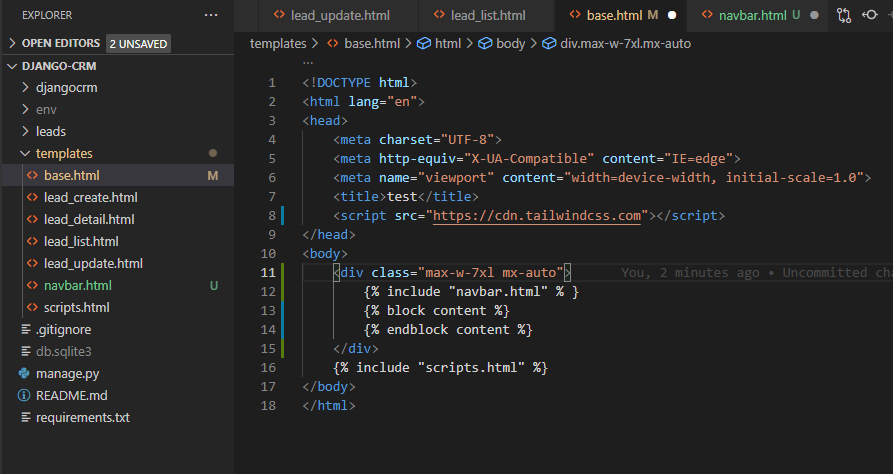
- now edit some buttons on navbar.html.
- edit/copy the below code
<header class="text-gray-600 body-font">
<div class="container mx-auto flex flex-wrap p-5 flex-col md:flex-row items-center">
<a class="flex title-font font-medium items-center text-gray-900 mb-4 md:mb-0">
<svg xmlns="http://www.w3.org/2000/svg" fill="none" stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" class="w-10 h-10 text-white p-2 bg-indigo-500 rounded-full" viewBox="0 0 24 24" data-darkreader-inline-stroke="" style="--darkreader-inline-stroke:currentColor;">
<path d="M12 2L2 7l10 5 10-5-10-5zM2 17l10 5 10-5M2 12l10 5 10-5"></path>
</svg>
<span class="ml-3 text-xl">DJ CRM</span>
</a>
<nav class="md:ml-auto flex flex-wrap items-center text-base justify-center">
<a class="mr-5 hover:text-gray-900">Signup</a>
</nav>
<button class="inline-flex items-center bg-gray-100 border-0 py-1 px-3 focus:outline-none hover:bg-gray-200 rounded text-base mt-4 md:mt-0">
Login
<svg fill="none" stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" class="w-4 h-4 ml-1" viewBox="0 0 24 24" data-darkreader-inline-stroke="" style="--darkreader-inline-stroke:currentColor;">
<path d="M5 12h14M12 5l7 7-7 7"></path>
</svg>
</button>
</div>
</header>
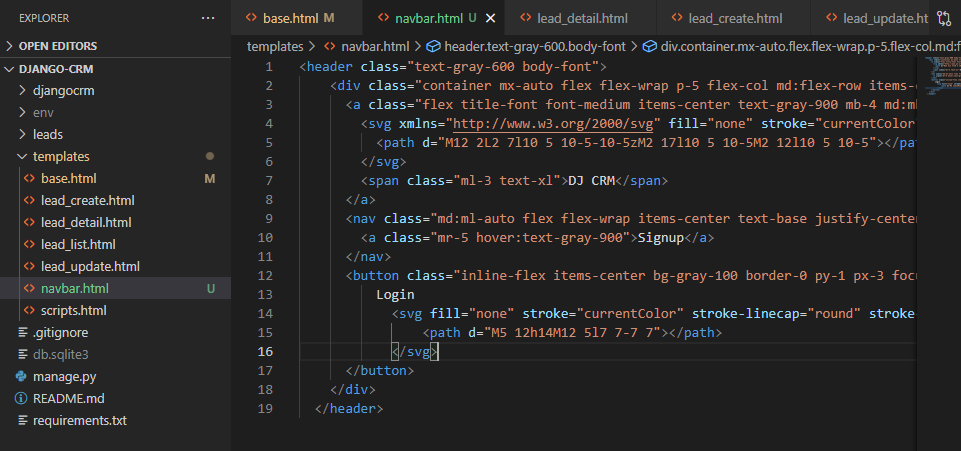
3. Using tailblocks to style landing page
- create landing.html file under templates
- write/copy the code below there
{% extends 'base.html' %}
{% block content %}
{% endblock content %}
- go to tailblocks and grab any code you like. in video and my case second of HERO
- copy them inside of block content
- edit some texts there
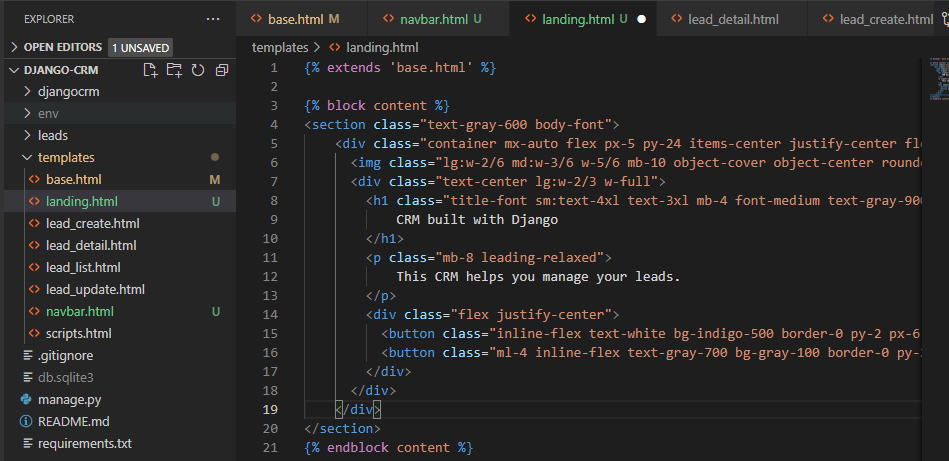
4. Add landing page on views and urls
- go to views.py under leads folder
- add function for landing page like below
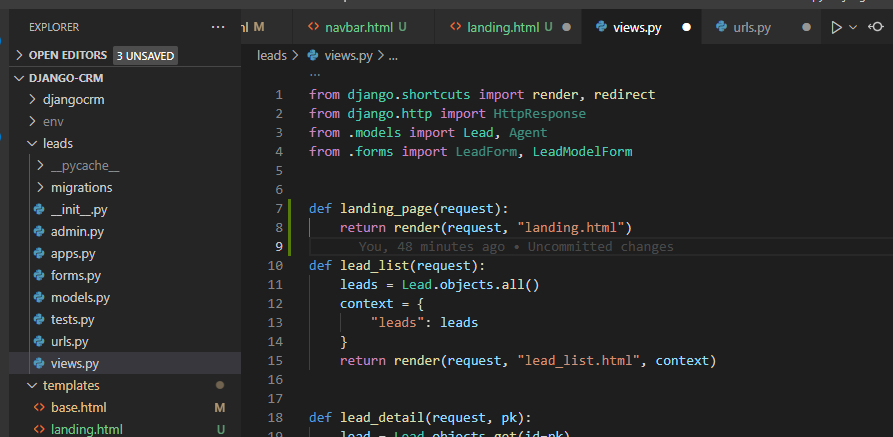
- go to urls.py under root project folder, in my case djangocrm folder
- add path for landing page like below
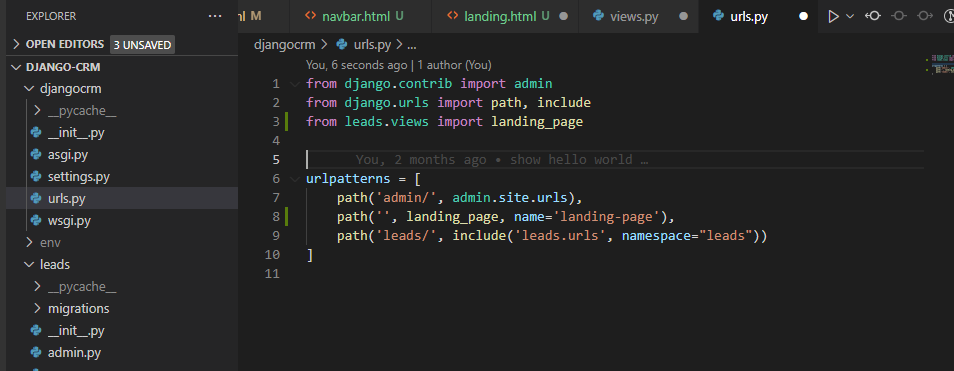
- save the files and go to browser to check on localhost:8000
- you will see the landing page there.
5. add lead button on navbar
- go to navbar.html
- write/copy code below
<a href="{% url 'leads:lead-list' %}"class="mr-5 hover:text-gray-900">Leads</a>
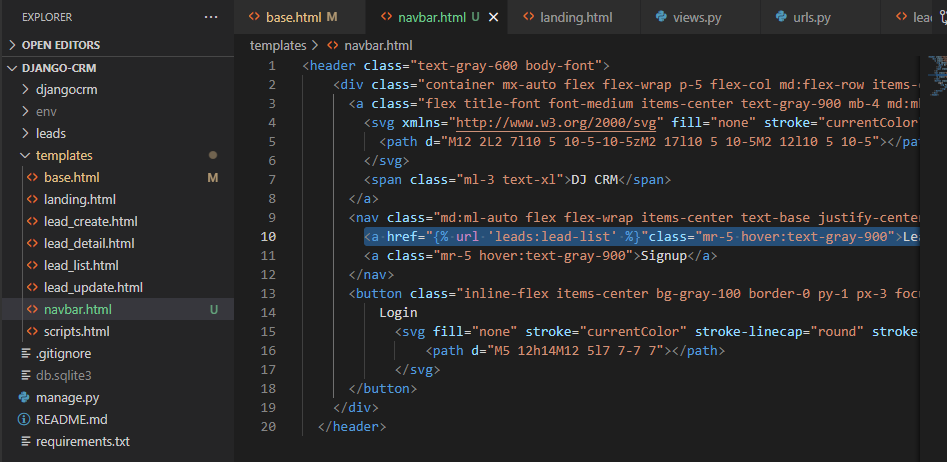
6. Styling lead_list page
- go to tailblocks, find the one you like. in video and my case, use fourth of FEATURE
- copy paste whole code inside of block content
- add/edit the ones we already have and adding some styles like below code
- I am not going over all the detail of css, for the description of each of them, please go to their site and watch the video.
{% extends "base.html" %}
{% block content %}
<section class="text-gray-600 body-font">
<div class="container px-5 py-24 mx-auto flex flex-wrap">
<div class="w-full mb-6 py-6 flex justify-between items-center border-b border-gray-200">
<div>
<h1 class="text-4xl text-gray-800">Leads</h1>
</div>
<div>
<a href="{% url 'leads:lead-create' %}" class="text-gray-500 hover:text-blue-500">
Create a new lead
</a>
</div>
</div>
<div class="flex flex-wrap -m-4">
{% for lead in leads %}
<div class="p-4 lg:w-1/2 md:w-full">
<div class="flex border-2 rounded-lg border-gray-200 border-opacity-50 p-8 sm:flex-row flex-col">
<div class="w-16 h-16 sm:mr-8 sm:mb-0 mb-4 inline-flex items-center justify-center rounded-full bg-indigo-100 text-indigo-500 flex-shrink-0">
<svg fill="none" stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" class="w-8 h-8" viewBox="0 0 24 24">
<path d="M22 12h-4l-3 9L9 3l-3 9H2"></path>
</svg>
</div>
<div class="flex-grow">
<h2 class="text-gray-900 text-lg title-font font-medium mb-3">
{{ lead.first_name }} {{ lead.last_name }}
</h2>
<p class="leading-relaxed text-base">
Blue bottle crucifix vinyl post-ironic four dollar toast vegan taxidermy. Gastropub indxgo juice poutine.
</p>
<a href="{% url 'leads:lead-detail' lead.pk %}" class="mt-3 text-indigo-500 inline-flex items-center">
View lead details
<svg fill="none" stroke="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" class="w-4 h-4 ml-2" viewBox="0 0 24 24">
<path d="M5 12h14M12 5l7 7-7 7"></path>
</svg>
</a>
</div>
</div>
</div>
{% endfor %}
</div>
</div>
</section>
{% endblock content %}
- the code is in mess format because of tabs, but if you copy and paste it on vs code, you will see ok in format. I hope…
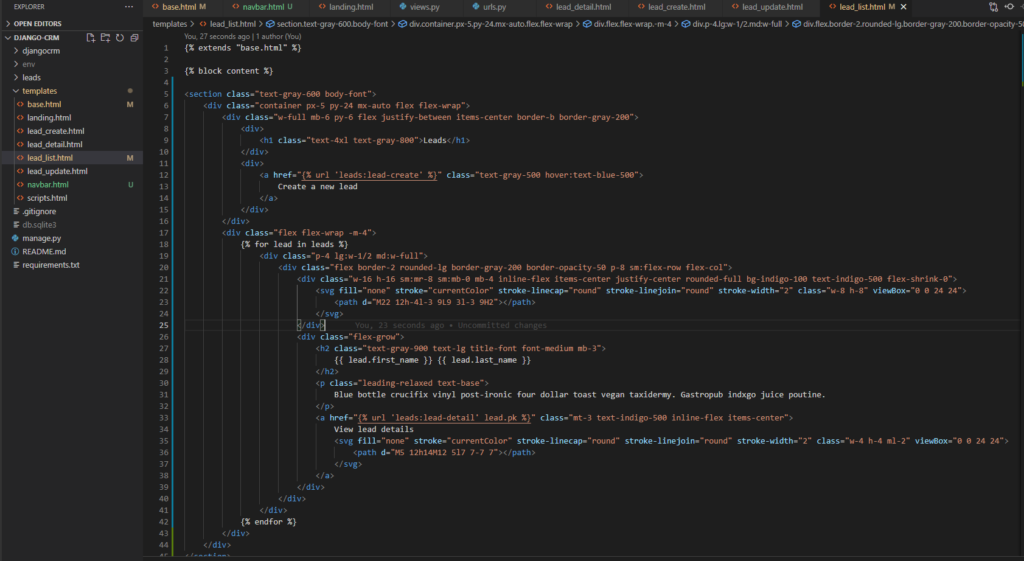
7. Styling lead detail view
- go to tailblocks, find the one you like. in video and my case, use third of ECOMMERCE
- copy paste whole code inside of block content
- add/edit the ones we already have and adding some styles like below code
- Again, I am not going over all the detail of css, for the description of each of them, please go to their site and watch the video.
{% extends "base.html" %}
{% block content %}
<section class="text-gray-600 body-font overflow-hidden">
<div class="container px-5 py-24 mx-auto">
<div class="lg:w-4/5 mx-auto flex flex-wrap">
<div class="lg:w-1/2 w-full lg:pr-10 lg:py-6 mb-6 lg:mb-0">
<h2 class="text-sm title-font text-gray-500 tracking-widest">
LEAD
</h2>
<h1 class="text-gray-900 text-3xl title-font font-medium mb-4">
{{ lead.first_name }} {{ lead.last_name }}
</h1>
<div class="flex mb-4">
<a class="flex-grow text-indigo-500 border-b-2 border-indigo-500 py-2 text-lg px-1">
Description
</a>
<a class="flex-grow border-b-2 border-gray-300 py-2 text-lg px-1">
Reviews
</a>
<a href="{% url 'leads:lead-update' lead.pk %}" class="flex-grow border-b-2 border-gray-300 py-2 text-lg px-1">
Details
</a>
</div>
<p class="leading-relaxed mb-4">
Fam locavore kickstarter distillery. Mixtape chillwave tumeric sriracha taximy chia microdosing tilde DIY. XOXO fam inxigo juiceramps cornhole raw denim forage brooklyn. Everyday carry +1 seitan poutine tumeric. Gastropub blue bottle austin listicle pour-over, neutra jean.
</p>
<div class="flex border-t border-gray-200 py-2">
<span class="text-gray-500">Age</span>
<span class="ml-auto text-gray-900">{{ lead.age }}</span>
</div>
<div class="flex border-t border-gray-200 py-2">
<span class="text-gray-500">Location</span>
<span class="ml-auto text-gray-900">Dummy Location</span>
</div>
<div class="flex border-t border-b mb-6 border-gray-200 py-2">
<span class="text-gray-500">Cell Phone</span>
<span class="ml-auto text-gray-900">+654651651</span>
</div>
<div class="flex">
<button class="flex ml-auto text-white bg-indigo-500 border-0 py-2 px-6 focus:outline-none hover:bg-indigo-600 rounded">Button</button>
<button class="rounded-full w-10 h-10 bg-gray-200 p-0 border-0 inline-flex items-center justify-center text-gray-500 ml-4">
<svg fill="currentColor" stroke-linecap="round" stroke-linejoin="round" stroke-width="2" class="w-5 h-5" viewBox="0 0 24 24">
<path d="M20.84 4.61a5.5 5.5 0 00-7.78 0L12 5.67l-1.06-1.06a5.5 5.5 0 00-7.78 7.78l1.06 1.06L12 21.23l7.78-7.78 1.06-1.06a5.5 5.5 0 000-7.78z"></path>
</svg>
</button>
</div>
</div>
<img alt="ecommerce" class="lg:w-1/2 w-full lg:h-auto h-64 object-cover object-center rounded" src="https://dummyimage.com/400x400">
</div>
</div>
</section>
{% endblock content %}
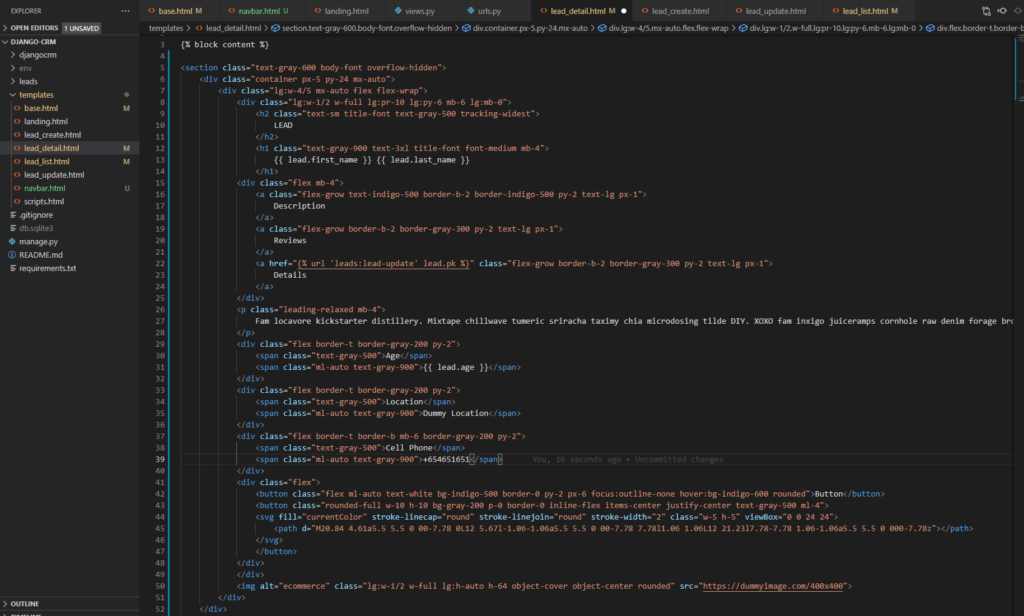
8. styling update view
- since we are using same page for update, copy the whole thing on detail page and paste on update.
- add/edit the ones we already have and adding some styles like below code
- Again, I am not going over all the detail of css, for the description of each of them, please go to their site and watch the video.
{% extends "base.html" %}
{% block content %}
<section class="text-gray-600 body-font overflow-hidden">
<div class="container px-5 py-24 mx-auto">
<div class="lg:w-4/5 mx-auto flex flex-wrap">
<div class="lg:w-1/2 w-full lg:pr-10 lg:py-6 mb-6 lg:mb-0">
<h2 class="text-sm title-font text-gray-500 tracking-widest">
LEAD
</h2>
<h1 class="text-gray-900 text-3xl title-font font-medium mb-4">
{{ lead.first_name }} {{ lead.last_name }}
</h1>
<div class="flex mb-4">
<a href="{% url 'leads:lead-detail' lead.pk %}" class="flex-grow border-b-2 border-gray-300 py-2 text-lg px-1">
Description
</a>
<a class="flex-grow border-b-2 border-gray-300 py-2 text-lg px-1">
Reviews
</a>
<a href="{% url 'leads:lead-update' lead.pk %}" class="flex-grow text-indigo-500 border-b-2 border-indigo-500 py-2 text-lg px-1">
Update Details
</a>
</div>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Submit</button>
</form>
<a href="{% url 'leads:lead-delete' lead.pk %}" class="w-1/2 mt-3 flex ml-auto text-white bg-indigo-500 border-0 py-2 px-6 focus:outline-none hover:bg-indigo-600 rounded">
Delete
</a>
</div>
<img alt="ecommerce" class="lg:w-1/2 w-full lg:h-auto h-64 object-cover object-center rounded" src="https://dummyimage.com/400x400">
</div>
</div>
</section>
{% endblock content %}
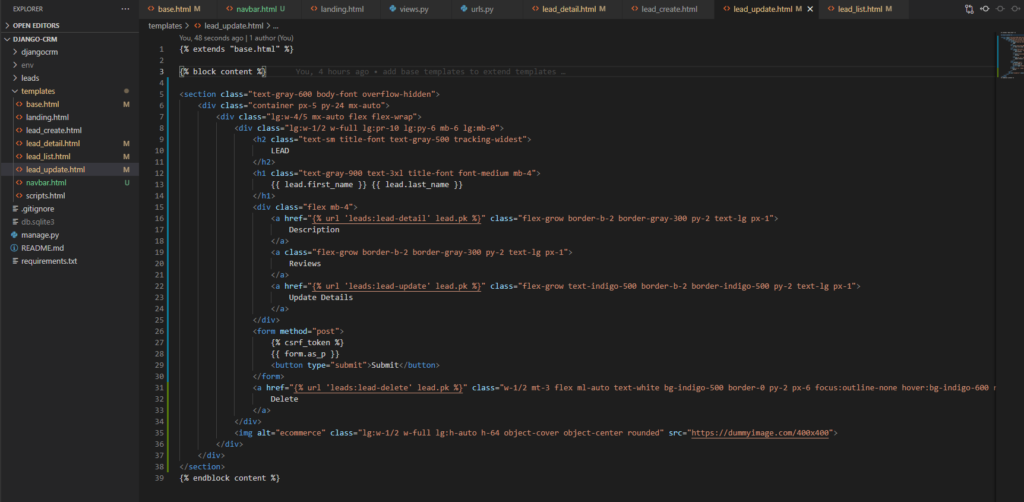
- save all the files and check on your browser.
- it is not perfectly done yet, but it will be done later.
done for this post