This post is for myself to remember how to build Django project.
I did follow the tutorial from the JustDjango Learn website free tutorial.
And this is the fourth tutorial named Getting Started With Django.
I am not going over all the details and descriptions for each part. There are good explanations on video of the JustDjango Learn. So, visit their site and try their tutorials if you need more details. Also, the orders of this post and their video might be different because I put things first what I think should come first.
I am doing this on Windows 10 with just Windows PowerShell. Not using virtual machines at all.
1. Login Required
- To make pages as login required, restrict from non-members
- go to views.py
- import LoginRequiredMixin
- add them to each of classes that needed
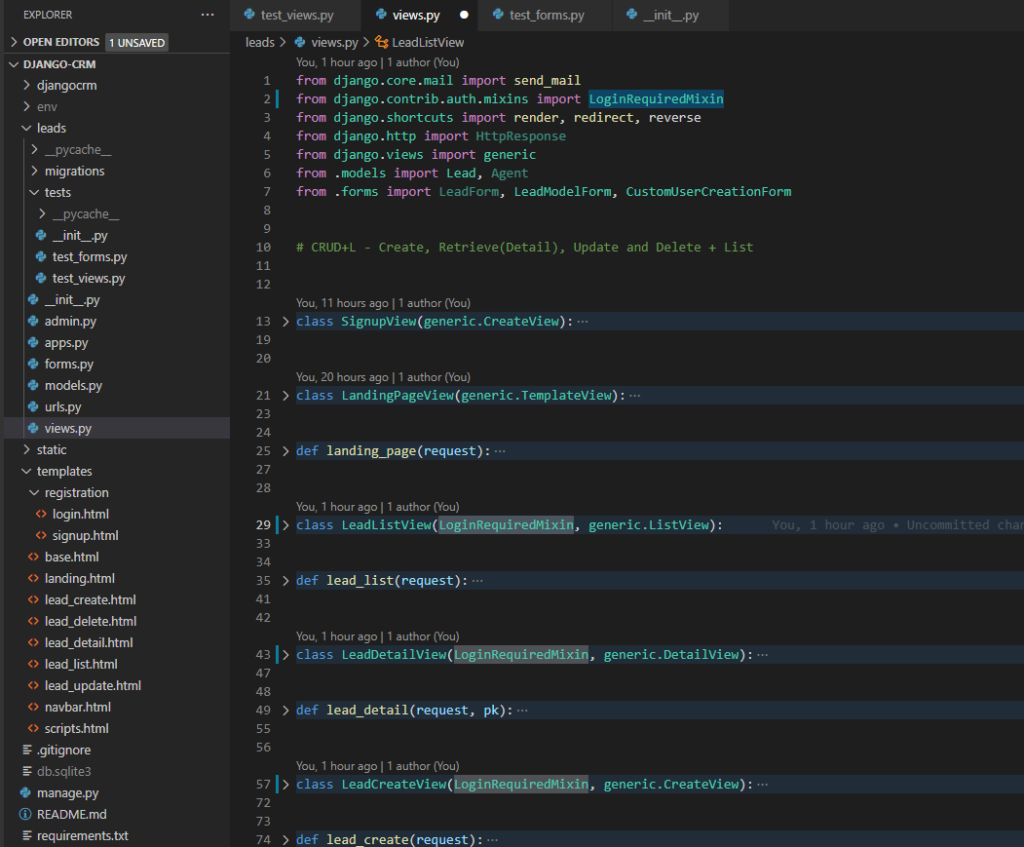
- save the file and go to browser
- if you are logged in, logout and direct to the leads or other pages that you added LoginRequiredMixin
- you will see the error like screenshot below
- that’s because you don’t have rights to access to the page.
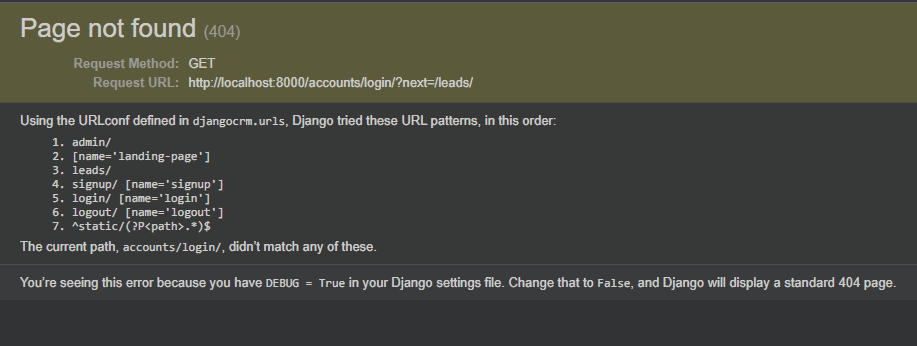
2. redirect to the login page
- instead show error page, will show login page when have no rights to access to the page.
- to do that, go to settings.py
- add below code at the bottom
LOGIN_URL = "/login"
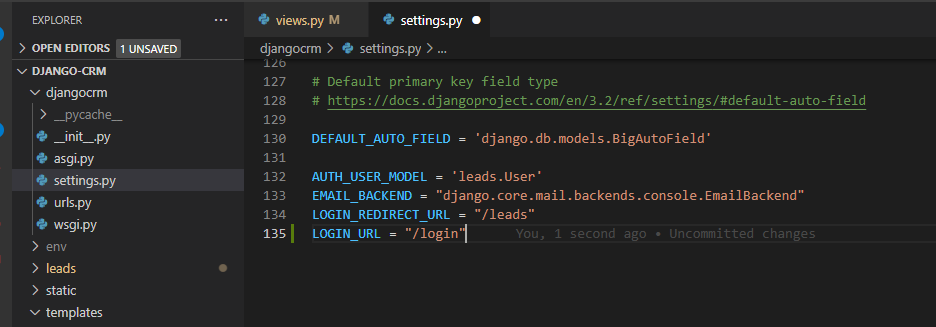
- save file, go to browser and go to leads or other pages to test
- you will see login page instead error
3. show right info(data) for each user
- currently, admin, test, or all the users can see all same info on pages
- need to give different rights/access to each users
- to do that, go to models.py under leads folder
- copy/write code below
- there were some missing function under Agent class, so I added to.
from django.db import models
from django.contrib.auth.models import AbstractUser
# Create your models here.
class User(AbstractUser):
pass
class UserProfile(models.Model):
user = models.OneToOneField(User, on_delete=models.CASCADE)
def __str__(self):
return self.user.username
class Lead(models.Model):
first_name = models.CharField(max_length=20)
last_name = models.CharField(max_length=20)
age = models.IntegerField(default=0)
agent = models.ForeignKey("Agent", on_delete=models.CASCADE)
class Agent(models.Model):
user = models.OneToOneField(User, on_delete=models.CASCADE)
organisation = models.ForeignKey(UserProfile, on_delete=models.CASCADE)
def __str__(self):
return self.user.email
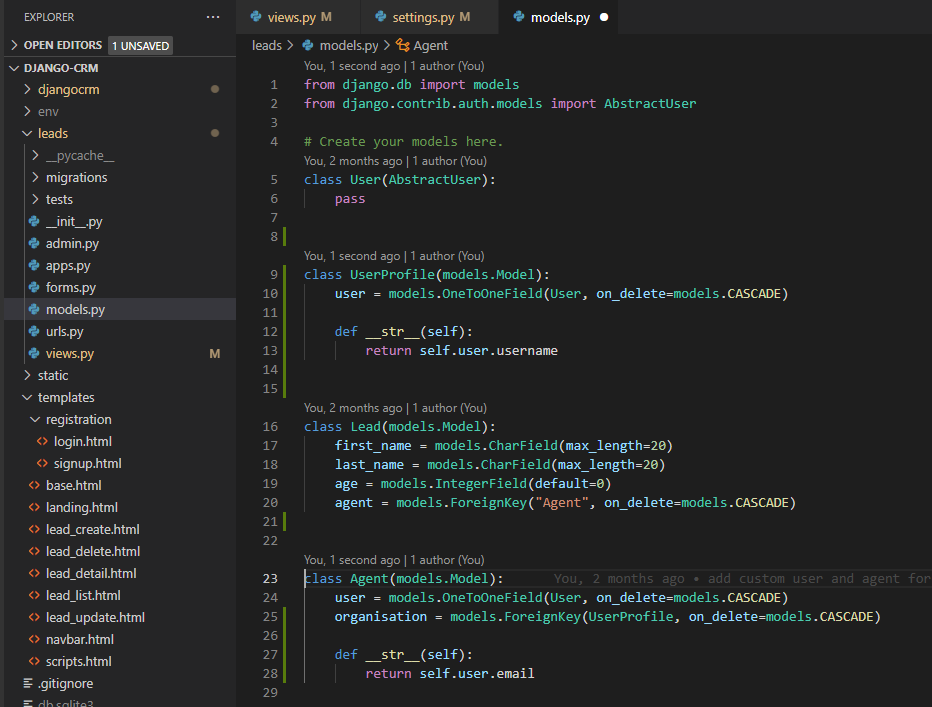
- go to admin.py under leads folder
- import UserProfile and add admin
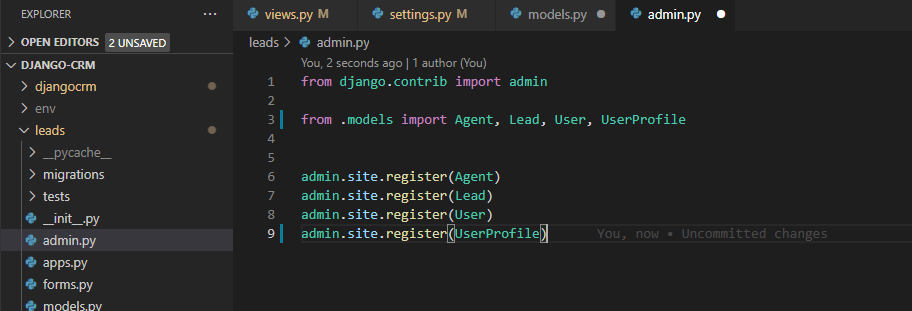
- stop the server
- make migrations
- you will get something like screenshot below

- select option 1
- then, you will get another message
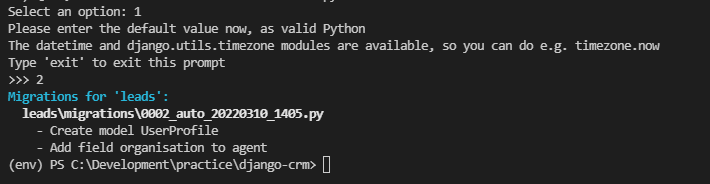
- then, type 1 or 2 or whatever.
- then, if you try migrate, you will see another message like below.

- or none. if nothing, but succeed migrate, don’t have to do any others,
- if get above message, delete db.sqlite3
- and migrate again
- for the description of why delete db, please see their video
- since the db is reset, need to create superuser again
- go to check user profiles is there or not on admin.
4. Signals
- signals can be used in all kinds of events
- currently, user profile is not created automatically when user is created.
- to make it happen, using django built-in signals
- go to models.py
- import post_save from signals
- write/copy below code at the bottom
def post_user_created_signal(sender, instance, created, **kwargs):
print(instance, created)
if created:
UserProfile.objects.create(user=instance)
post_save.connect(post_user_created_signal, sender=User)
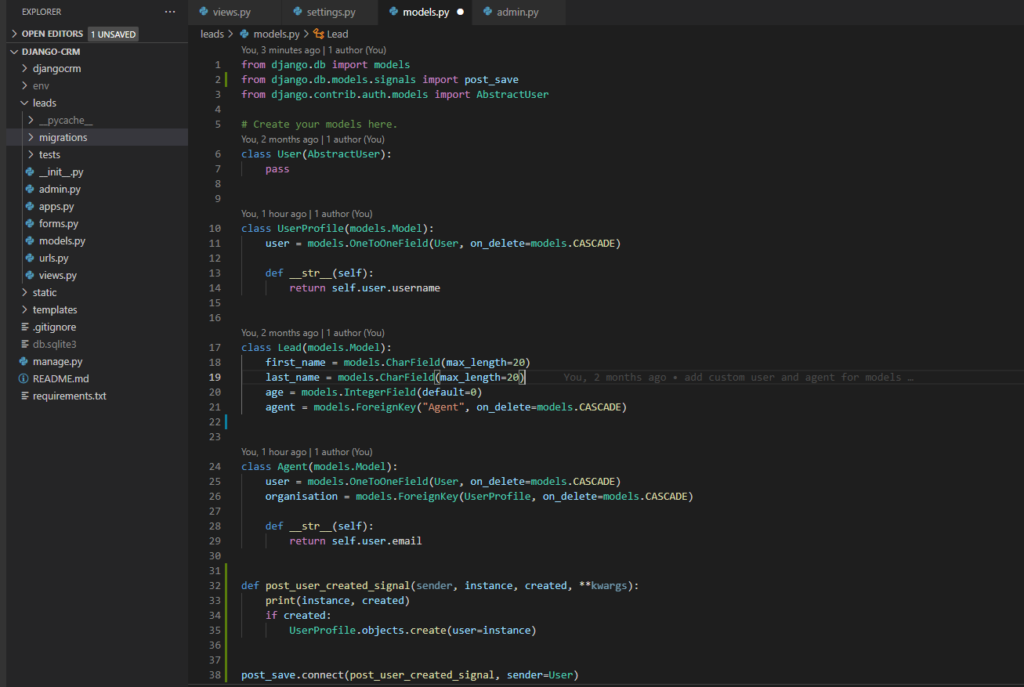
- save the file and go to admin page to try to create new user to test
- you will see user profiles is created for newly created user.
done for this post